JavaScript Learning Resources
JavaScript is one of the most fundamental technologies on the World Wide Web and a cornerstone of web development. It is widely used for adding interactivity and dynamic behavior to web pages, making it an indispensable part of modern web applications. JavaScript plays a pivotal role in enhancing user experiences and enabling real-time interactions on the internet. Its applications span from creating interactive elements on basic websites to powering complex web applications and services.
Resources:
1. Introduction to JavaScript
- What is JavaScript
- History
- Uses
- Integration with HTML and CSS
Resources:
- YouTube: Programming with Mosh - JavaScript Tutorial for Beginners
- Website: MDN Web Docs - JavaScript Basics
2. JavaScript Fundamentals
- Variables
- Data types
- Operators
- Comments
- Basic input/output
Resources:
3. Control Structures
- Conditionals (if, else, switch)
- Loops (for, while, do-while)
- Logical operators
Resources:
4. Functions in JavaScript
- Defining functions
- Function expressions
- Arrow functions
- Scope
Resources:
5. Arrays and Objects
- Array methods
- Iterating over arrays
- Objects
- Object methods
- this keyword
Resources:
- YouTube: Academind - JavaScript Arrays and Objects
- Website: MDN Web Docs - JavaScript Arrays
- Website: MDN Web Docs - JavaScript Objects
6. DOM Manipulation
- Selecting elements
- Modifying elements
- Event listeners
- Creating elements
Resources:
7. Events in JavaScript
- Event types (click, input, etc.)
- Event handling
- Event bubbling
- Event delegation
Resources:
8. Asynchronous JavaScript
- Callbacks
- Promises
- Async/await
- AJAX
Resources:
9. Error Handling
- Try-catch
- Throwing errors
- Custom error handling
Resources:
10. ES6 and Modern JavaScript
- Let/const
- Arrow functions
- Template literals
- Destructuring
- Spread/rest
- Modules
Resources:
11. Object-Oriented Programming (OOP)
- Classes
- Constructors
- Inheritance
- Encapsulation
- Prototypes
Resources:
12. JavaScript Patterns and Best Practices
- Design patterns (module, singleton, factory)
- DRY principle
- Writing clean code
Resources:
13. Advanced Topics: Closures and Prototypes
- Closures
- Currying
- Higher-order functions
- Prototype inheritance
Resources:
14. JavaScript Frameworks (React, Vue, Angular)
- Introduction to popular frameworks
- Basic setup
- Use cases
Resources:
15. JavaScript Testing
- Unit testing
- Jest
- Mocha
- Testing best practices
Resources:
16. Working with APIs
- Fetch API
- JSON
- Working with external APIs
Resources:
17. JavaScript Projects and Challenges
- Building projects like To-Do apps, weather apps, etc.
Resources:
Download Free JavaScript Notes Now with CoderStar!
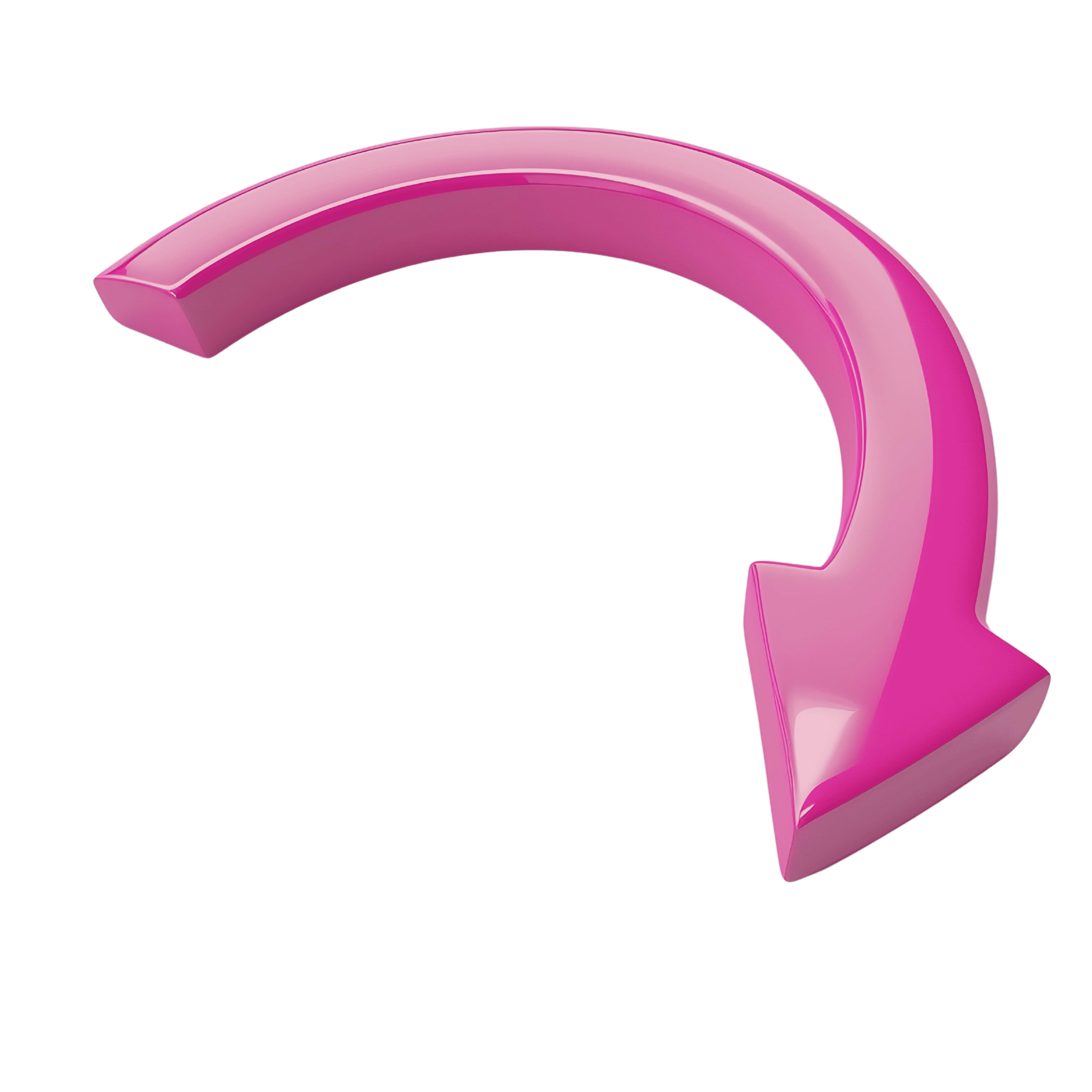
"Your generous contribution to CoderStar not only fuels innovation but also empowers the coding community to reach new heights. Thank you for being a key part of our journey!"