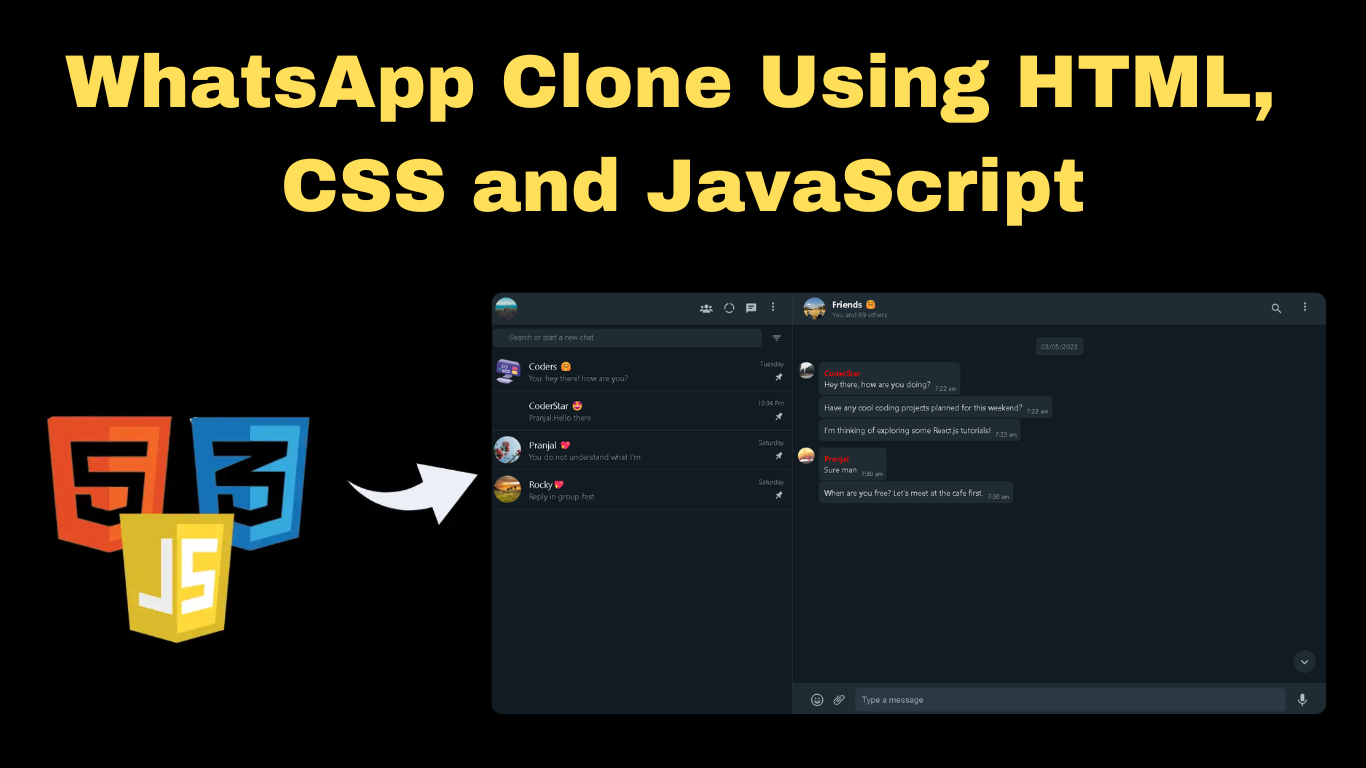
Building a WhatsApp Web UI Clone with HTML, CSS, and JavaScript
Introduction
The WhatsApp Web UI clone project is designed to replicate the user interface of the popular messaging platform, WhatsApp Web. This project focuses on implementing key UI components, such as sidebars, chat windows, and message elements, using only HTML, CSS, and JavaScript. While the emphasis is on creating a visually similar layout and style, it also introduces basic interactivity to enhance the user experience.
Project Features
The WhatsApp Web Clone includes the following features:
- Sidebar Navigation: Includes a profile section, toolbar icons, search bar, and a list of chats.
- Dynamic Chat Window: Displays chat messages with a scrollable layout and input tools for sending messages.
- Responsive Design: Adapts seamlessly to different screen sizes and devices.
- Interactive Elements: Hover effects, scroll-to-top functionality, and connectivity notifications.
Project Output-
1.Landing Page:
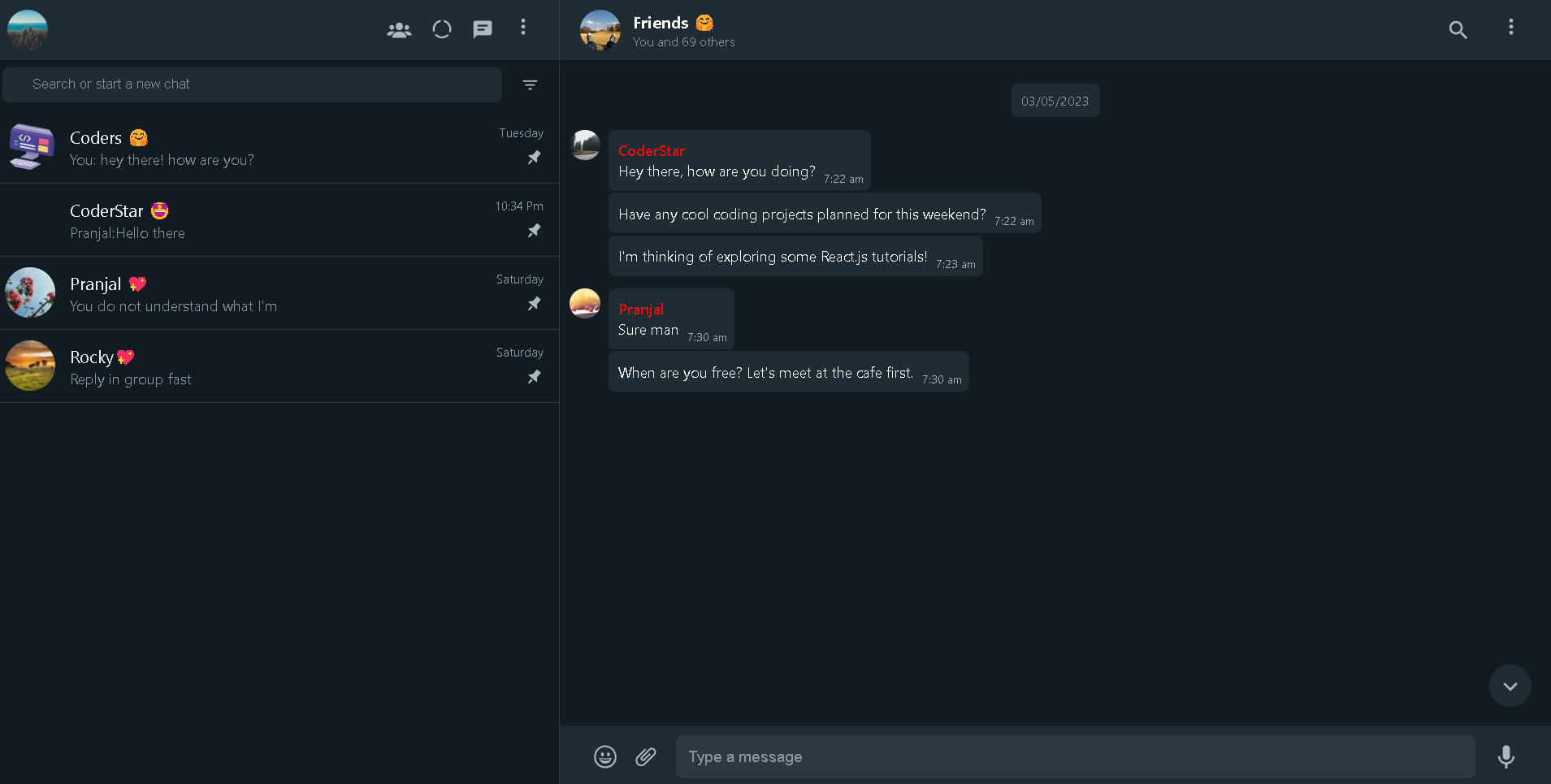
2. Settings Option
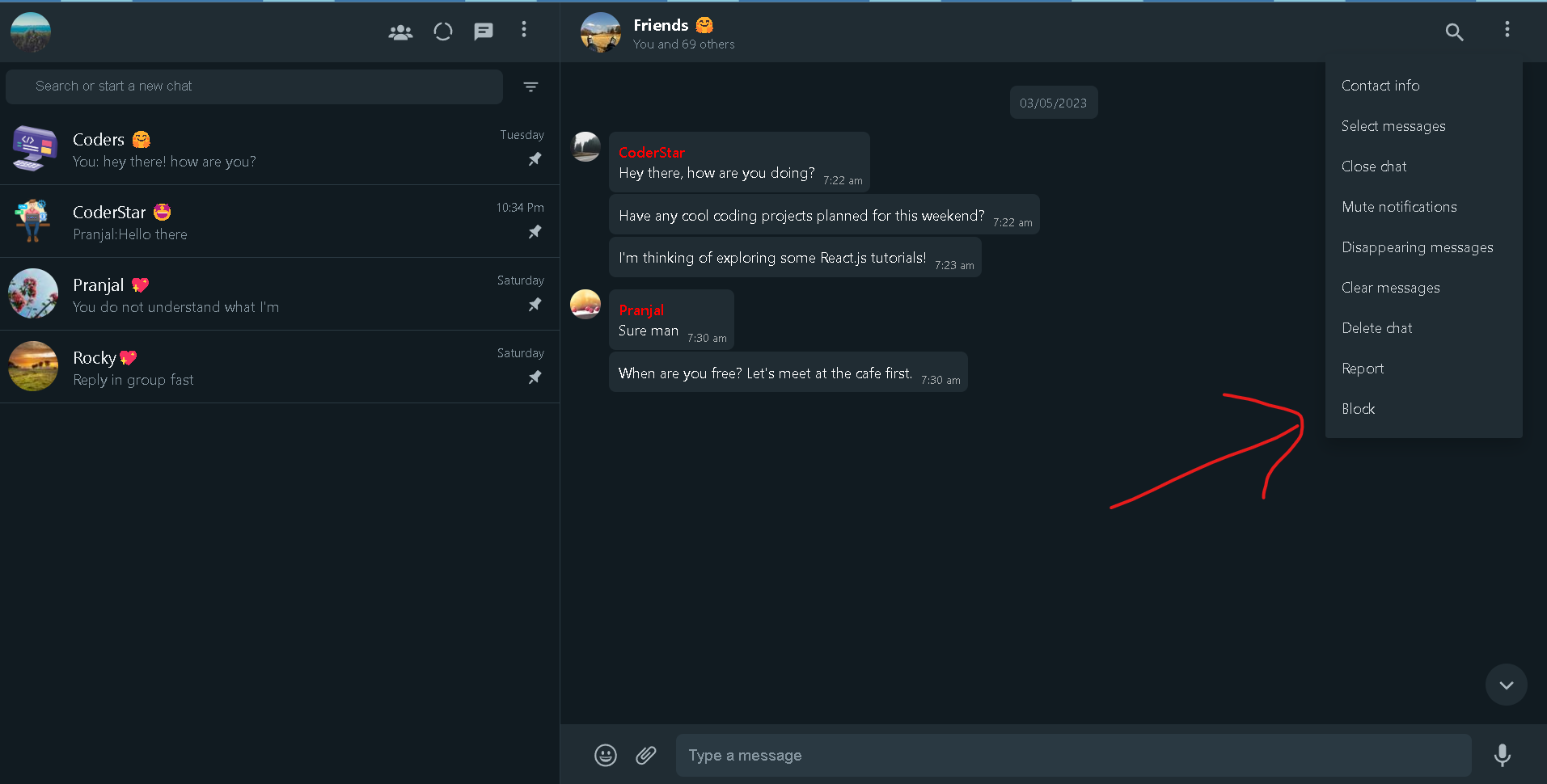
3. Sidebar Settings Option
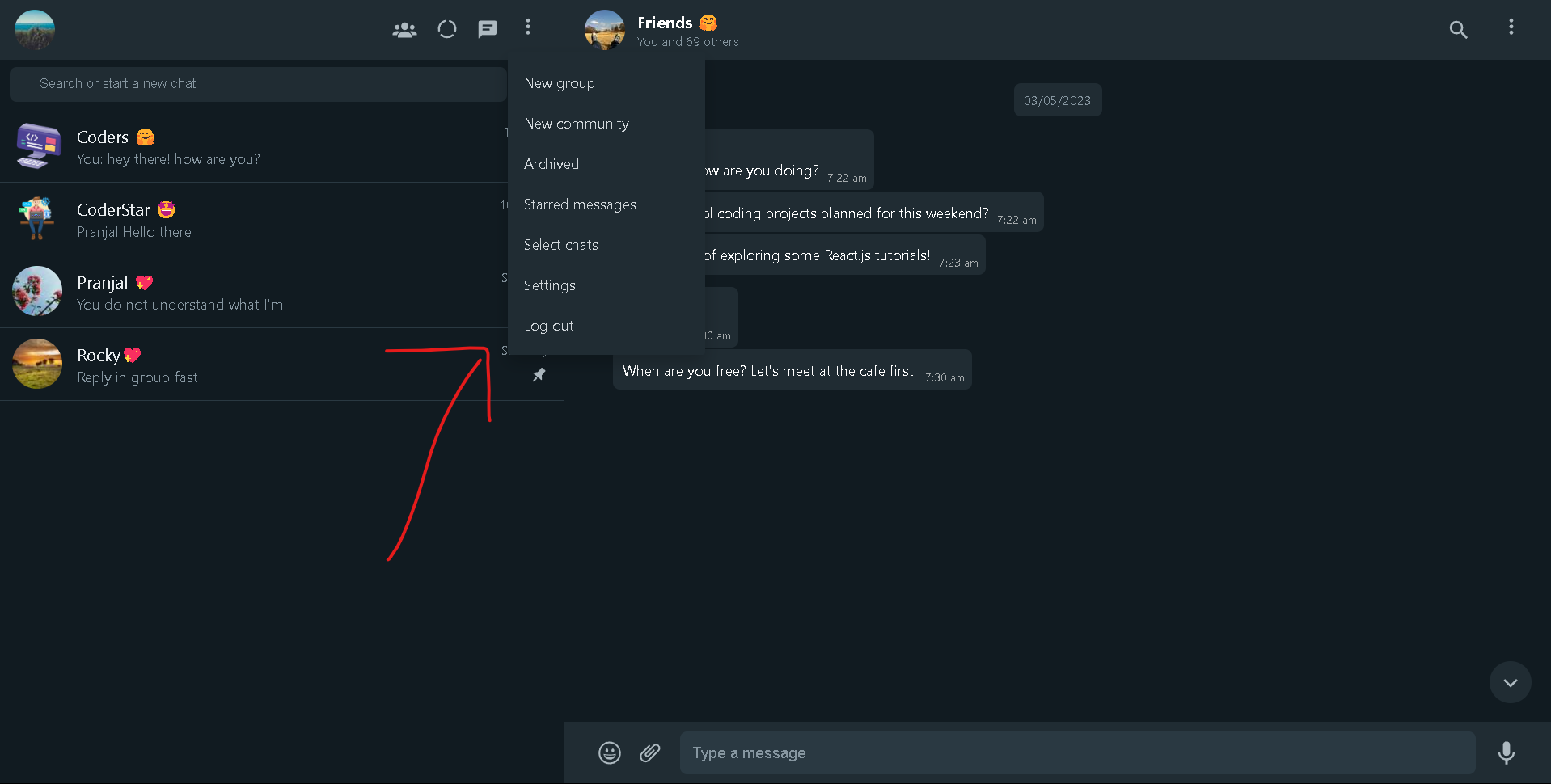
Project Structure
HTML Layout
here, the project is structured into two main sections:
- Sidebar: Displays user information, shortcuts, and chat lists.
- Chat Window: The primary area for conversations, displaying messages and providing interaction tools.
CSS Styling
To replicate Youtube’s signature design, CSS (Cascading Style Sheets) is used extensively.
- Global Styles:
- Resetting margins, paddings, and box-sizing for consistency.
- Defining fonts and base colors for a cohesive theme.
- Sidebar:
- Utilizes flexbox for vertical alignment.
- Stylish hover effects for interactivity.
- Chat Window:
- Chat bubbles styled with rounded corners and shadow effects.
- Scrollable content area for smooth navigation.
JavaScript Interactivity
The primary focus of this project is on replicating the UI, with JavaScript providing minor enhancements:
Key Interactivity Features:
- Connectivity Notification:
- navigator.onLine: Detects the online/offline status of the browser
- Event Listeners
- online: When the browser reconnects, the notification is hidden.
- offline: When the browser loses connectivity, the notification is displayed.
- Class Manipulation
- Adds or removes the hidden class to toggle the visibility of the notification.
- Scroll-to-Top Functionality:
const connectivityNotification = document.getElementsByClassName( "connectivity-notification" ) [0]; connectivityNotification.classList.add("hidden"); window.addEventListener("online", () => { connectivityNotification.classList.add("hidden"); console.log(connectivityNotification.classList); console.log("online"); }); window.addEventListener("offline", () => { connectivityNotification.classList.remove("hidden"); console.log("offline"); });
Explanation
The Scroll-to-Top Functionality allows users to quickly navigate to the top of the chat window. This feature is implemented using JavaScript to detect scroll positions and handle button visibility and interactions.
const chatWindowContents = document.getElementById("chat-window-contents"); const scrollToTopButton = document.getElementsByClassName( "scroll-to-top-button" )[0]; const scrollToTopButtonIcon = document.getElementsByClassName( "scroll-to-top-button-icon" )[0]; chatWindowContents.addEventListener("scroll", (event) => { const scrollPositionFromTop = chatWindowContents.scrollTop; const scrollFromBottom = chatWindowContents.scrollHeight - scrollPositionFromTop - chatWindowContents.clientHeight; if (scrollFromBottom === 0) { scrollToTopButton.classList.add("shrink"); scrollToTopButtonIcon.classList.add("shrink"); } else { scrollToTopButton.classList.remove("shrink"); scrollToTopButtonIcon.classList.remove("shrink"); } }); scrollToTopButton.addEventListener("click", (event) => { event.preventDefault(); chatWindowContents.scrollTop = chatWindowContents.scrollHeight - chatWindowContents.clientHeight; });
Explanation
- Scroll Detection:
- The scroll event listener on the chat window detects the user's scrolling behavior.
- The scroll position is calculated to determine whether the button should be visible.
- Dynamic Button Behavior:
- Show Button: When the user scrolls up, the button becomes visible by removing the shrink class.
- Hide Button: When the user scrolls to the bottom, the button is hidden by adding the shrink class.
- Smooth Scrolling:
- Clicking the button triggers a scroll to the top of the chat window for a smooth navigation experience.
Benefits
- Enhances navigation in long conversations.
- Improves usability by providing a quick and intuitive way to scroll to the top.
Conclusion
This WhatsApp Web UI clone demonstrates how to construct a visually appealing and responsive user interface with HTML, CSS, and JavaScript. By breaking the project into smaller components like the sidebar and chat window, developers can learn essential principles of web design and front-end development. Start building your own WhatsApp Web UI clone today to sharpen your skills and deepen your understanding of modern web development!
WhatsApp Clone Project Source Code Download Here:
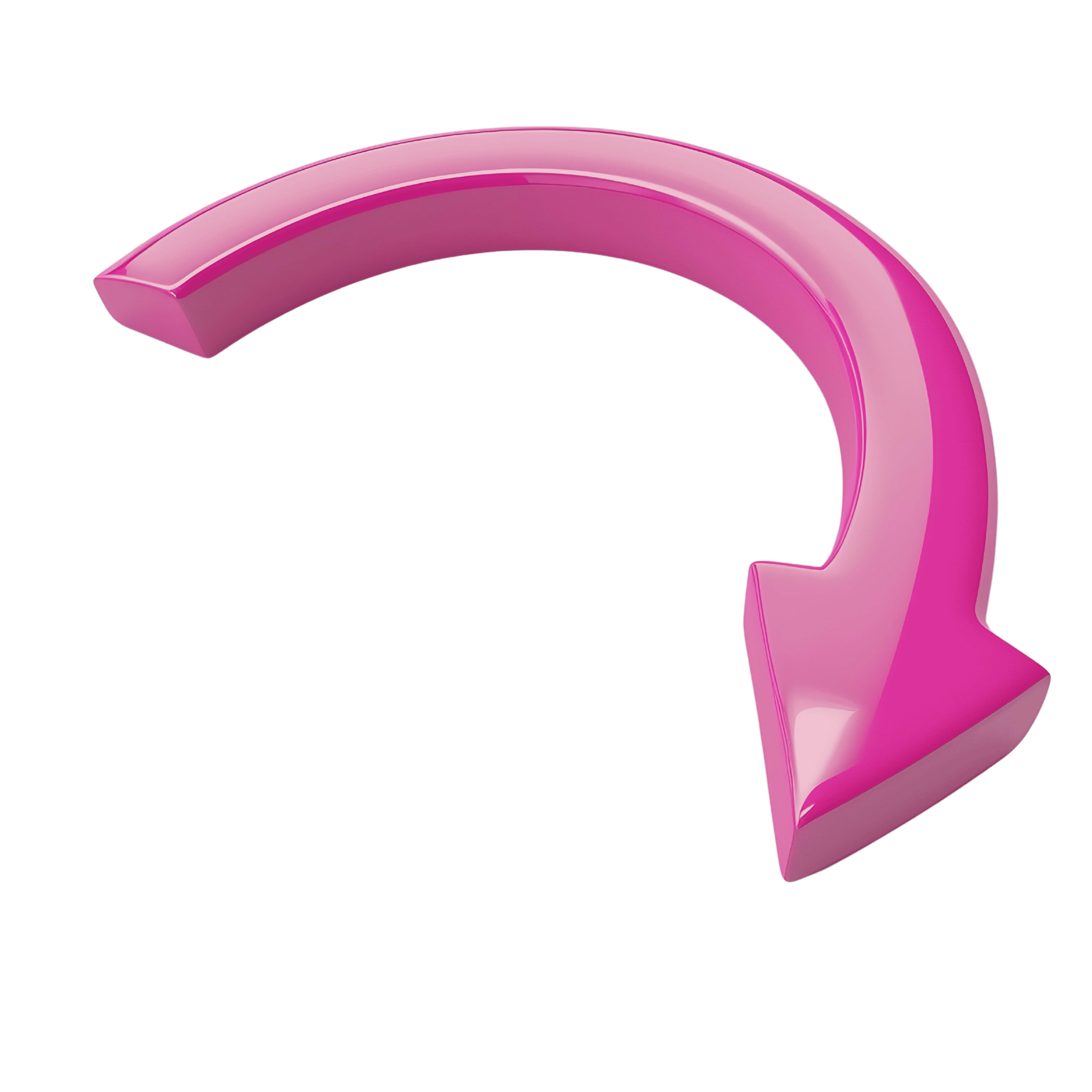
"Your generous contribution to CoderStar not only fuels innovation but also empowers the coding community to reach new heights. Thank you for being a key part of our journey!"